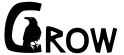 |
Crow
1.0
A C++ microframework for the web
|
|
|
enum | HTTPMethod : char {
HTTPMethod::DELETE = 0,
HTTPMethod::GET,
HTTPMethod::HEAD,
HTTPMethod::POST,
HTTPMethod::PUT,
HTTPMethod::CONNECT,
HTTPMethod::OPTIONS,
HTTPMethod::TRACE,
HTTPMethod::PATCH,
HTTPMethod::PURGE,
HTTPMethod::COPY,
HTTPMethod::LOCK,
HTTPMethod::MKCOL,
HTTPMethod::MOVE,
HTTPMethod::PROPFIND,
HTTPMethod::PROPPATCH,
HTTPMethod::SEARCH,
HTTPMethod::UNLOCK,
HTTPMethod::BIND,
HTTPMethod::REBIND,
HTTPMethod::UNBIND,
HTTPMethod::ACL,
HTTPMethod::REPORT,
HTTPMethod::MKACTIVITY,
HTTPMethod::CHECKOUT,
HTTPMethod::MERGE,
HTTPMethod::MSEARCH,
HTTPMethod::NOTIFY,
HTTPMethod::SUBSCRIBE,
HTTPMethod::UNSUBSCRIBE,
HTTPMethod::MKCALENDAR,
HTTPMethod::LINK,
HTTPMethod::UNLINK,
HTTPMethod::SOURCE,
HTTPMethod::Delete = 0,
HTTPMethod::Get,
HTTPMethod::Head,
HTTPMethod::Post,
HTTPMethod::Put,
HTTPMethod::Connect,
HTTPMethod::Options,
HTTPMethod::Trace,
HTTPMethod::Patch,
HTTPMethod::Purge,
HTTPMethod::Copy,
HTTPMethod::Lock,
HTTPMethod::MkCol,
HTTPMethod::Move,
HTTPMethod::Propfind,
HTTPMethod::Proppatch,
HTTPMethod::Search,
HTTPMethod::Unlock,
HTTPMethod::Bind,
HTTPMethod::Rebind,
HTTPMethod::Unbind,
HTTPMethod::Acl,
HTTPMethod::Report,
HTTPMethod::MkActivity,
HTTPMethod::Checkout,
HTTPMethod::Merge,
HTTPMethod::MSearch,
HTTPMethod::Notify,
HTTPMethod::Subscribe,
HTTPMethod::Unsubscribe,
HTTPMethod::MkCalendar,
HTTPMethod::Link,
HTTPMethod::Unlink,
HTTPMethod::Source,
HTTPMethod::InternalMethodCount
} |
|
enum | status {
CONTINUE = 100,
SWITCHING_PROTOCOLS = 101,
OK = 200,
CREATED = 201,
ACCEPTED = 202,
NON_AUTHORITATIVE_INFORMATION = 203,
NO_CONTENT = 204,
RESET_CONTENT = 205,
PARTIAL_CONTENT = 206,
MULTIPLE_CHOICES = 300,
MOVED_PERMANENTLY = 301,
FOUND = 302,
SEE_OTHER = 303,
NOT_MODIFIED = 304,
TEMPORARY_REDIRECT = 307,
PERMANENT_REDIRECT = 308,
BAD_REQUEST = 400,
UNAUTHORIZED = 401,
FORBIDDEN = 403,
NOT_FOUND = 404,
METHOD_NOT_ALLOWED = 405,
PROXY_AUTHENTICATION_REQUIRED = 407,
CONFLICT = 409,
GONE = 410,
PAYLOAD_TOO_LARGE = 413,
UNSUPPORTED_MEDIA_TYPE = 415,
RANGE_NOT_SATISFIABLE = 416,
EXPECTATION_FAILED = 417,
PRECONDITION_REQUIRED = 428,
TOO_MANY_REQUESTS = 429,
UNAVAILABLE_FOR_LEGAL_REASONS = 451,
INTERNAL_SERVER_ERROR = 500,
NOT_IMPLEMENTED = 501,
BAD_GATEWAY = 502,
SERVICE_UNAVAILABLE = 503,
GATEWAY_TIMEOUT = 504,
VARIANT_ALSO_NEGOTIATES = 506
} |
|
enum | ParamType : char {
ParamType::INT,
ParamType::UINT,
ParamType::DOUBLE,
ParamType::STRING,
ParamType::PATH,
ParamType::MAX
} |
|
enum | LogLevel {
LogLevel::DEBUG = 0,
LogLevel::INFO,
LogLevel::WARNING,
LogLevel::ERROR,
LogLevel::CRITICAL,
LogLevel::Debug = 0,
LogLevel::Info,
LogLevel::Warning,
LogLevel::Error,
LogLevel::Critical
} |
|
|
const std::string | crlf ("\r\n") |
|
std::string | method_name (HTTPMethod method) |
|
template<typename T > |
const std::string & | get_header_value (const T &headers, const std::string &key) |
| Find and return the value associated with the key. (returns an empty string if nothing is found) More...
|
|
int | qs_strncmp (const char *s, const char *qs, size_t n) |
|
int | qs_parse (char *qs, char *qs_kv[], int qs_kv_size) |
|
int | qs_decode (char *qs) |
|
char * | qs_k2v (const char *key, char *const *qs_kv, int qs_kv_size, int nth) |
|
char * | qs_scanvalue (const char *key, const char *qs, char *val, size_t val_len) |
|
boost::optional< std::pair< std::string, std::string > > | qs_dict_name2kv (const char *dict_name, char *const *qs_kv, int qs_kv_size, int nth=0) |
|
static std::string | base64encode (const unsigned char *data, size_t size, const char *key="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/") |
|
static std::string | base64encode (std::string data, size_t size, const char *key="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/") |
|
static std::string | base64encode_urlsafe (const unsigned char *data, size_t size) |
|
static std::string | base64encode_urlsafe (std::string data, size_t size) |
|
static std::string | base64decode (const char *data, size_t size) |
|
static std::string | base64decode (const std::string &data, size_t size) |
|
static std::string | base64decode (const std::string &data) |
|
static void | sanitize_filename (std::string &data, char replacement='_') |
|
◆ App
template<typename... Middlewares>
◆ SimpleApp
◆ ci_map
◆ tcp
◆ HTTPMethod
Enumerator |
---|
DELETE | |
GET | |
HEAD | |
POST | |
PUT | |
CONNECT | |
OPTIONS | |
TRACE | |
PATCH | |
PURGE | |
COPY | |
LOCK | |
MKCOL | |
MOVE | |
PROPFIND | |
PROPPATCH | |
SEARCH | |
UNLOCK | |
BIND | |
REBIND | |
UNBIND | |
ACL | |
REPORT | |
MKACTIVITY | |
CHECKOUT | |
MERGE | |
MSEARCH | |
NOTIFY | |
SUBSCRIBE | |
UNSUBSCRIBE | |
MKCALENDAR | |
LINK | |
UNLINK | |
SOURCE | |
Delete | |
Get | |
Head | |
Post | |
Put | |
Connect | |
Options | |
Trace | |
Patch | |
Purge | |
Copy | |
Lock | |
MkCol | |
Move | |
Propfind | |
Proppatch | |
Search | |
Unlock | |
Bind | |
Rebind | |
Unbind | |
Acl | |
Report | |
MkActivity | |
Checkout | |
Merge | |
MSearch | |
Notify | |
Subscribe | |
Unsubscribe | |
MkCalendar | |
Link | |
Unlink | |
Source | |
InternalMethodCount | |
◆ status
Enumerator |
---|
CONTINUE | |
SWITCHING_PROTOCOLS | |
OK | |
CREATED | |
ACCEPTED | |
NON_AUTHORITATIVE_INFORMATION | |
NO_CONTENT | |
RESET_CONTENT | |
PARTIAL_CONTENT | |
MULTIPLE_CHOICES | |
MOVED_PERMANENTLY | |
FOUND | |
SEE_OTHER | |
NOT_MODIFIED | |
TEMPORARY_REDIRECT | |
PERMANENT_REDIRECT | |
BAD_REQUEST | |
UNAUTHORIZED | |
FORBIDDEN | |
NOT_FOUND | |
METHOD_NOT_ALLOWED | |
PROXY_AUTHENTICATION_REQUIRED | |
CONFLICT | |
GONE | |
PAYLOAD_TOO_LARGE | |
UNSUPPORTED_MEDIA_TYPE | |
RANGE_NOT_SATISFIABLE | |
EXPECTATION_FAILED | |
PRECONDITION_REQUIRED | |
TOO_MANY_REQUESTS | |
UNAVAILABLE_FOR_LEGAL_REASONS | |
INTERNAL_SERVER_ERROR | |
NOT_IMPLEMENTED | |
BAD_GATEWAY | |
SERVICE_UNAVAILABLE | |
GATEWAY_TIMEOUT | |
VARIANT_ALSO_NEGOTIATES | |
◆ ParamType
Enumerator |
---|
INT | |
UINT | |
DOUBLE | |
STRING | |
PATH | |
MAX | |
◆ LogLevel
Enumerator |
---|
DEBUG | |
INFO | |
WARNING | |
ERROR | |
CRITICAL | |
Debug | |
Info | |
Warning | |
Error | |
Critical | |
◆ crlf()
const std::string crow::crlf |
( |
"\r\n" |
| ) |
|
◆ method_name()
std::string crow::method_name |
( |
HTTPMethod |
method | ) |
|
|
inline |
◆ get_header_value()
template<typename T >
const std::string& crow::get_header_value |
( |
const T & |
headers, |
|
|
const std::string & |
key |
|
) |
| |
|
inline |
Find and return the value associated with the key. (returns an empty string if nothing is found)
◆ qs_strncmp()
int crow::qs_strncmp |
( |
const char * |
s, |
|
|
const char * |
qs, |
|
|
size_t |
n |
|
) |
| |
|
inline |
◆ qs_parse()
int crow::qs_parse |
( |
char * |
qs, |
|
|
char * |
qs_kv[], |
|
|
int |
qs_kv_size |
|
) |
| |
|
inline |
◆ qs_decode()
int crow::qs_decode |
( |
char * |
qs | ) |
|
|
inline |
◆ qs_k2v()
char * crow::qs_k2v |
( |
const char * |
key, |
|
|
char *const * |
qs_kv, |
|
|
int |
qs_kv_size, |
|
|
int |
nth = 0 |
|
) |
| |
|
inline |
◆ qs_scanvalue()
char * crow::qs_scanvalue |
( |
const char * |
key, |
|
|
const char * |
qs, |
|
|
char * |
val, |
|
|
size_t |
val_len |
|
) |
| |
|
inline |
◆ qs_dict_name2kv()
boost::optional<std::pair<std::string, std::string> > crow::qs_dict_name2kv |
( |
const char * |
dict_name, |
|
|
char *const * |
qs_kv, |
|
|
int |
qs_kv_size, |
|
|
int |
nth = 0 |
|
) |
| |
|
inline |
◆ base64encode() [1/2]
static std::string crow::base64encode |
( |
const unsigned char * |
data, |
|
|
size_t |
size, |
|
|
const char * |
key = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/" |
|
) |
| |
|
inlinestatic |
◆ base64encode() [2/2]
static std::string crow::base64encode |
( |
std::string |
data, |
|
|
size_t |
size, |
|
|
const char * |
key = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/" |
|
) |
| |
|
inlinestatic |
◆ base64encode_urlsafe() [1/2]
static std::string crow::base64encode_urlsafe |
( |
const unsigned char * |
data, |
|
|
size_t |
size |
|
) |
| |
|
inlinestatic |
◆ base64encode_urlsafe() [2/2]
static std::string crow::base64encode_urlsafe |
( |
std::string |
data, |
|
|
size_t |
size |
|
) |
| |
|
inlinestatic |
◆ base64decode() [1/3]
static std::string crow::base64decode |
( |
const char * |
data, |
|
|
size_t |
size |
|
) |
| |
|
inlinestatic |
◆ base64decode() [2/3]
static std::string crow::base64decode |
( |
const std::string & |
data, |
|
|
size_t |
size |
|
) |
| |
|
inlinestatic |
◆ base64decode() [3/3]
static std::string crow::base64decode |
( |
const std::string & |
data | ) |
|
|
inlinestatic |
◆ sanitize_filename()
static void crow::sanitize_filename |
( |
std::string & |
data, |
|
|
char |
replacement = '_' |
|
) |
| |
|
inlinestatic |
◆ cr
const char crow::cr = '\r' |
◆ lf
const char crow::lf = '\n' |
◆ method_strings
constexpr const char* crow::method_strings[] |
|
constexpr |
◆ mime_types
const std::unordered_map<std::string, std::string> crow::mime_types |
◆ INVALID_BP_ID
constexpr const uint16_t crow::INVALID_BP_ID {((uint16_t)-1)} |
|
constexpr |
◆ RULE_SPECIAL_REDIRECT_SLASH
const int crow::RULE_SPECIAL_REDIRECT_SLASH = 1 |
◆ VERSION
constexpr const char crow::VERSION[] = "master" |
|
constexpr |
std::string header_field
Definition: parser.h:181
bool has(const std::string &str) const
Definition: json.h:495
response & operator=(const response &r)=delete
virtual const char * what() const
Definition: mustache.h:26
const routing_params & params
Definition: routing.h:112
void operator()(F &&f) const
Definition: middleware.h:312
@ CREATED
Definition: common.h:173
std::string url
Definition: parser.h:178
response(response &&r)
Definition: http_response.h:88
void post(CompletionHandler handler)
Send data through the socket and return immediately.
Definition: websocket.h:117
const rvalue & operator[](const std::string &str) const
Definition: json.h:586
void set_default_timeout(std::uint8_t timeout)
Set the default timeout for this task_timer instance. (Default: 5)
Definition: task_timer.h:83
unsigned char http_ver_major
Definition: http_request.h:33
request(HTTPMethod method, std::string raw_url, std::string url, query_string url_params, ci_map headers, std::string body, unsigned char http_major, unsigned char http_minor, bool has_keep_alive, bool has_close_connection, bool is_upgrade)
Construct a request with all values assigned.
Definition: http_request.h:46
uint32_t mask_
Definition: websocket.h:626
self_t & signal_add(int signal_number)
Definition: app.h:111
char * qs_k2v(const char *key, char *const *qs_kv, int qs_kv_size, int nth)
Definition: query_string.h:172
PerMethod()
Definition: routing.h:1598
const std::string & rule()
Definition: routing.h:80
std::function< void(crow::websocket::connection &, const std::string &)> close_handler_
Definition: websocket.h:636
wvalue(std::string &&value)
Definition: json.h:1311
static LogLevel get_current_log_level()
Definition: logging.h:127
void reset()
Definition: json.h:1438
query_string(std::string url)
Definition: query_string.h:334
@ PROXY_AUTHENTICATION_REQUIRED
Definition: common.h:193
wvalue & operator[](const std::string &str)
Definition: json.h:1674
CORSRules & blueprint(const Blueprint &bp)
Handle CORS for a specific blueprint.
Definition: cors.h:154
void stop()
Stop the server.
Definition: app.h:319
Trie trie
Definition: routing.h:1595
type
Definition: json.h:79
size_t get_size()
Definition: routing.h:994
std::string body
Definition: http_request.h:31
void * middleware_container
Definition: http_request.h:37
boost::array< char, 4096 > buffer_
Definition: http_connection.h:603
void foreach_method(F f)
Definition: routing.h:68
Definition: middleware.h:261
void set_header_no_override(const std::string &key, const std::string &value, crow::response &res)
Set header key to value if it is not set.
Definition: cors.h:97
std::chrono::steady_clock clock_type
Definition: task_timer.h:24
task_timer(boost::asio::io_service &io_service)
Definition: task_timer.h:28
void cancel_deadline_timer()
Definition: http_connection.h:578
std::enable_if<!is_before_handle_arity_3_impl< MW >::value >::type before_handler_call(MW &mw, request &req, response &res, Context &ctx, ParentContext &)
Definition: middleware.h:97
bool has_handler()
Definition: routing.h:354
std::string msg
Definition: mustache.h:30
Definition: middleware.h:64
Definition: middleware.h:300
bool is_binary_
Definition: websocket.h:617
void context
Definition: socket_adaptors.h:20
const char * end() const
Definition: json.h:166
std::unordered_map< std::string, wvalue > object
Definition: json.h:1242
request to_request() const
Take the parsed HTTP request data and convert it to a crow::request.
Definition: parser.h:172
handler_type_helper< ArgsWrapped... >::type handler_
Definition: routing.h:253
wvalue & operator=(double value)
Definition: json.h:1460
Definition: cookie_parser.h:31
r_string(r_string &&r)
Definition: json.h:136
static int on_headers_complete(http_parser *self_)
Definition: parser.h:66
Action(ActionType t, size_t start, size_t end, size_t pos=0)
Definition: mustache.h:66
Route * tptr
Definition: middleware.h:318
tcp::socket & socket()
Get the object handling data transfers, this can be either a TCP socket or an SSL stream (if SSL is e...
Definition: socket_adaptors.h:37
bool close_connection
Definition: http_request.h:34
std::unordered_map< std::string, std::string > jar
Definition: cookie_parser.h:35
query_string url_params
The parameters associated with the request. (everything after the ?)
Definition: http_request.h:29
void after_handle(request &, response &res, context &ctx)
Definition: cookie_parser.h:98
virtual std::string get_remote_ip()=0
bool is_empty()
Check whether or not the trie is empty.
Definition: routing.h:657
char * pop(const std::string &name)
Works similar to get() except it removes the item from the query string.
Definition: query_string.h:376
rvalue & operator=(rvalue &&r) noexcept
Definition: json.h:264
std::vector< char * > get_list(const std::string &name, bool use_brackets=true) const
Returns a list of values, passed as ?name[]=value1&name[]=value2&...name[]=valuen with n being the si...
Definition: query_string.h:398
uint16_t pick_io_service_idx()
Definition: http_server.h:180
void validate()
Definition: routing.h:738
std::vector< std::string > keys()
Definition: json.h:633
@ SWITCHING_PROTOCOLS
Definition: common.h:170
ci_map headers
Definition: http_request.h:30
const char * begin() const
Definition: json.h:165
void set_cached() const
Definition: json.h:653
@ SEE_OTHER
Definition: common.h:183
std::unordered_multimap< std::string, std::string, ci_hash, ci_key_eq > ci_map
Definition: ci_map.h:35
LogLevel level_
Definition: logging.h:145
void set_static_file_info(std::string path)
Return a static file as the response body.
Definition: http_response.h:227
wvalue & operator=(list &&v)
Definition: json.h:1557
friend std::enable_if<(N==0)>::type after_handlers_call_helper(Container &middlewares, Context &ctx, request &req, response &res)
Definition: middleware.h:163
void add_list_item(std::string &list, const std::string &val)
build comma separated list
Definition: cors.h:89
boost::asio::io_service & get_io_service()
Definition: socket_adaptors.h:25
An HTTP connection.
Definition: http_connection.h:34
void set_(Func f, typename std::enable_if< std::is_same< typename std::tuple_element< 0, std::tuple< Args..., void >>::type, const request & >::value &&!std::is_same< typename std::tuple_element< 1, std::tuple< Args..., void, void >>::type, response & >::value, int >::type=0)
Definition: routing.h:209
virtual void log(std::string message, LogLevel level)=0
int64_t si
Definition: json.h:1255
char * e_
End.
Definition: json.h:173
Definition: middleware.h:32
void escape(const std::string &str, std::string &ret)
Definition: json.h:39
Local middleware should extend ILocalMiddleware.
Definition: middleware.h:16
void notify_server_start()
Notify anything using wait_for_server_start() to proceed.
Definition: app.h:269
@ BAD_GATEWAY
Definition: common.h:206
friend std::ostream & operator<<(std::ostream &os, const r_string &s)
Definition: json.h:175
std::tuple< uint16_t, std::vector< uint16_t >, routing_params > find(const std::string &req_url, const Node *node=nullptr, unsigned pos=0, routing_params *params=nullptr, std::vector< uint16_t > *blueprints=nullptr) const
Definition: routing.h:746
std::string message_
Definition: websocket.h:618
void wait_for_server_start()
Wait until the server has properly started.
Definition: app.h:426
std::string fragment_
Definition: websocket.h:619
@ NOT_MODIFIED
Definition: common.h:184
self_t & onaccept(Func f)
Definition: routing.h:429
response(int code, returnable &value)
Definition: http_response.h:81
@ GATEWAY_TIMEOUT
Definition: common.h:208
std::string methods_
Definition: cors.h:118
void operator()(F cparams)
Definition: routing.h:134
void validate()
A wrapper for validate() in the router.
Definition: app.h:235
bool is_close_handler_called_
Definition: websocket.h:632
bool has_sent_close_
Definition: websocket.h:628
std::string path
Definition: http_response.h:221
void set_loader(std::function< std::string(std::string)> loader)
Definition: mustache.h:689
request req_
Definition: http_connection.h:606
void parse_section_head(std::string &lines, part &part)
Definition: multipart.h:205
Adaptor adaptor_
Definition: http_connection.h:600
void start(F f)
Definition: socket_adaptors.h:77
@ FOUND
Definition: common.h:182
request()
Construct an empty request. (sets the method to GET)
Definition: http_request.h:41
std::string key
Definition: routing.h:636
void check_destroy()
Destroy the Connection.
Definition: websocket.h:600
const std::unordered_map< std::string, std::string > mime_types
Definition: mime_types.h:7
std::function< void()> task_type
Definition: task_timer.h:20
Adaptor::context * adaptor_ctx_
Definition: http_server.h:247
response(int code)
Definition: http_response.h:67
std::string bindaddr_
Definition: app.h:440
std::condition_variable cv_started_
Definition: app.h:465
bool has_recv_close_
Definition: websocket.h:629
std::function< bool()> is_alive_helper_
Definition: http_response.h:269
T::context & get()
Definition: middleware_context.h:51
std::string name_
Definition: routing.h:86
static constexpr bool value
Definition: middleware.h:92
void do_write_sync(std::vector< asio::const_buffer > &buffers)
Definition: http_connection.h:549
bool is_alive()
Check if the connection is still alive (usually by checking the socket status).
Definition: http_response.h:204
std::vector< boost::asio::const_buffer > buffers_
Definition: http_connection.h:612
void shutdown_write()
Definition: socket_adaptors.h:64
This constains metadata (coming from the stat command) related to any static files associated with th...
Definition: http_response.h:219
std::ostringstream stringstream_
Definition: logging.h:144
std::unordered_multimap< std::string, header, ci_hash, ci_key_eq > mph_map
Multipart header map (key is header key).
Definition: multipart.h:31
template_t(std::string body)
Definition: mustache.h:76
bool has(const char *str) const
Check if the json object has the passed string as a key.
Definition: json.h:490
std::false_type call_global
Definition: middleware.h:18
int qs_parse(char *qs, char *qs_kv[], int qs_kv_size)
Definition: query_string.h:99
rvalue(rvalue &&r) noexcept
Definition: json.h:248
void redirect(const std::string &location)
Return a "Temporary Redirect" response.
Definition: http_response.h:136
std::tuple< Middlewares... > middlewares_
Definition: app.h:452
ci_map headers
HTTP headers.
Definition: http_response.h:39
CORSRules & headers(const std::string &header)
Set Access-Control-Allow-Headers. Default is "*".
Definition: cors.h:39
static LogLevel & get_log_level_ref()
Definition: logging.h:131
type t_
The type of the value.
Definition: json.h:1250
void send_text(const std::string &msg) override
Send a plaintext message.
Definition: websocket.h:162
ActionType t
Definition: mustache.h:65
std::string s
Value if type is a string.
Definition: json.h:1268
void operator()(std::string name, Func &&f)
Definition: routing.h:589
Definition: http_server.h:26
void register_blueprint(Blueprint &blueprint)
Definition: routing.h:1215
uint16_t rule_index
Definition: routing.h:633
std::function< std::string()> & get_cached_date_str
Definition: http_connection.h:629
CORSRules & blueprint(const Blueprint &bp)
Handle CORS for specific blueprint.
Definition: cors.h:190
void operator()(Func &&f)
Definition: routing.h:575
std::vector< part > parts
The individual parts of the message.
Definition: multipart.h:78
@ PARTIAL_CONTENT
Definition: common.h:178
void set_static_file_info_unsafe(std::string path)
Return a static file as the response body without sanitizing the path (use set_static_file_info inste...
Definition: http_response.h:234
rvalue * end() const
Definition: json.h:534
std::function< std::string(std::string)> & get_loader_ref()
Definition: mustache.h:660
query_string()
Definition: query_string.h:296
@ SERVICE_UNAVAILABLE
Definition: common.h:207
A rule that can change its parameters during runtime.
Definition: routing.h:480
Definition: mustache.h:60
@ VARIANT_ALSO_NEGOTIATES
Definition: common.h:209
Equals function for ci_map (unordered_multimap).
Definition: ci_map.h:27
bool validated_
Definition: app.h:438
std::string url_
Definition: query_string.h:484
self_t & signal_clear()
Definition: app.h:105
Adaptor adaptor_
Definition: websocket.h:611
@ GONE
Definition: common.h:195
void validate() override
Definition: routing.h:487
Definition: middleware.h:40
void get_found_bp(std::vector< uint16_t > &bp_i, std::vector< Blueprint * > &blueprints, std::vector< Blueprint * > &found_bps, uint16_t index=0)
Definition: routing.h:1373
void unescape() const
Convert escaped string character to their original form ("\\n" -> ' ').
Definition: json.h:425
self_t & loglevel(LogLevel level)
Set the server's log level.
Definition: app.h:176
asio::io_service io_service_
Definition: http_server.h:226
union crow::json::wvalue::number num
Value if type is a number.
Blueprint(const std::string &prefix, const std::string &static_dir)
Definition: routing.h:1035
void validate() override
Definition: routing.h:380
void determine_num_type()
Determines num_type from the string.
Definition: json.h:688
@ UNSUPPORTED_MEDIA_TYPE
Definition: common.h:197
rendered_template render() const
Output a returnable template from this mustache template.
Definition: mustache.h:360
double d() const
The double precision floating-point number value.
Definition: json.h:378
std::string server_name_
Definition: http_server.h:237
uint16_t port_
Definition: http_server.h:238
wvalue & operator=(const std::string &str)
Definition: json.h:1549
std::unique_ptr< object > o
Value if type is a JSON object.
Definition: json.h:1270
void before_handle(request &req, response &res, context &ctx)
Definition: cookie_parser.h:52
void prepare_buffers()
Definition: http_connection.h:272
wvalue(std::int64_t value)
Definition: json.h:1298
const detail::r_string & key() const
Definition: json.h:543
Blueprint(const std::string &prefix, const std::string &static_dir, const std::string &templates_dir)
Definition: routing.h:1038
void process_message()
Definition: parser.h:151
Handler * handler_
This is currently an HTTP connection object (crow::Connection).
Definition: parser.h:189
void add(const std::string &url, uint16_t rule_index, unsigned bp_prefix_length=0, uint16_t blueprint_index=INVALID_BP_ID)
Definition: routing.h:909
std::vector< std::unique_ptr< asio::io_service > > io_service_pool_
Definition: http_server.h:227
void * userdata_
Definition: websocket.h:36
struct stat statbuf
Definition: http_response.h:222
identifier_type schedule(const task_type &task)
Schedule the given task to be executed after the default amount of ticks.
Definition: task_timer.h:51
void do_read()
Definition: http_connection.h:473
wvalue(std::uint16_t value)
Definition: json.h:1285
std::string content_length_
Definition: http_connection.h:614
self_t & server_name(std::string server_name)
Set the server name.
Definition: app.h:139
response & res
Definition: routing.h:114
const std::string & get_header_value(const std::string &key) const
Definition: http_request.h:55
std::uint8_t timeout_
Definition: app.h:435
void validate() override
Definition: routing.h:566
query_string(const query_string &qs)
Definition: query_string.h:301
static void sanitize_filename(std::string &data, char replacement='_')
Definition: utility.h:671
Crow< Middlewares... > App
Definition: app.h:469
int qs_decode(char *qs)
Definition: query_string.h:143
tcp::acceptor acceptor_
Definition: http_server.h:230
decltype(std::declval< Adaptor >().raw_socket()) & socket()
The TCP socket on top of which the connection is established.
Definition: http_connection.h:75
size_t operator()(const std::string &key) const
Definition: ci_map.h:12
boost::asio::deadline_timer deadline_timer_
Definition: task_timer.h:125
void operator()(F cparams)
Definition: routing.h:124
CORSRules(CORSHandler *handler)
Definition: cors.h:85
logger(LogLevel level)
Definition: logging.h:96
self_t & multithreaded()
Run the server on multiple threads using all available threads.
Definition: app.h:153
static std::string base64encode_urlsafe(const unsigned char *data, size_t size)
Definition: utility.h:575
std::string get_boundary(const std::string &header) const
Definition: multipart.h:151
bool keep_alive
Whether or not the server should send a connection: Keep-Alive header to the client.
Definition: parser.h:186
logger & operator<<(T const &value)
Definition: logging.h:111
bool skip_body
Whether this is a response to a HEAD request.
Definition: http_response.h:44
CORSHandler * handler_
Definition: cors.h:123
self_t & stream_threshold(size_t threshold)
Set the response body size (in bytes) beyond which Crow automatically streams responses (Default is 1...
Definition: app.h:186
std::array< PerMethod, static_cast< int >HTTPMethod::InternalMethodCount)> per_methods_
Definition: routing.h:1601
void end(const std::string &body_part)
Same as end() except it adds a body part right before ending.
Definition: http_response.h:197
@ NOT_IMPLEMENTED
Definition: common.h:205
std::vector< std::atomic< unsigned int > > task_queue_length_pool_
Definition: http_server.h:240
const std::string & server_name_
Definition: http_connection.h:611
void handle(request &req, response &res)
Definition: routing.h:1445
wvalue & operator=(wvalue &&r)
Definition: json.h:1422
void handle_upgrade(const request &req, response &res, Adaptor &&adaptor)
Definition: routing.h:1301
SocketAdaptor(boost::asio::io_service &io_service, context *)
Definition: socket_adaptors.h:21
bool feed(const char *buffer, int length)
Parse a buffer into the different sections of an HTTP request.
Definition: parser.h:104
std::string body
Definition: parser.h:185
part parse_section(std::string §ion)
Definition: multipart.h:192
ActionType
Definition: mustache.h:49
Definition: routing.h:109
part get_part_by_name(const std::string &name)
Definition: multipart.h:86
WebSocketReadState
Definition: websocket.h:12
void handle_fragment()
Process the payload fragment.
Definition: websocket.h:482
std::function< void(const crow::request &, crow::response &, Args...)> type
Definition: routing.h:249
message(const ci_map &headers, const std::string &boundary, const std::vector< part > §ions)
Default constructor using default values.
Definition: multipart.h:126
bool done()
Definition: parser.h:124
bool close_connection_
Definition: websocket.h:623
int count(const std::string &str)
Definition: json.h:521
const std::string & get_header_value(const std::string &key)
Definition: http_response.h:60
wvalue & operator=(unsigned short value)
Definition: json.h:1469
static std::string base64encode(const unsigned char *data, size_t size, const char *key="ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/")
Definition: utility.h:534
rendered_template render(context &ctx) const
Apply the values from the context provided and output a returnable template from this mustache templa...
Definition: mustache.h:372
Definition: middleware.h:43
void clear()
Used for compatibility, same as reset()
Definition: json.h:1433
num_type nt_
Definition: json.h:715
void force(char *s, uint32_t length)
Definition: json.h:182
void shutdown_read()
Definition: socket_adaptors.h:70
void cancel(identifier_type id)
Definition: task_timer.h:38
std::tuple< Middlewares... > * middlewares_
Definition: http_connection.h:626
response(returnable &&value)
Definition: http_response.h:71
std::string pad(std::string &string, const char &padding='"') const
Definition: multipart.h:258
constexpr const uint16_t INVALID_BP_ID
Definition: routing.h:23
std::unordered_map< std::string, std::string > cookies_to_add
Definition: cookie_parser.h:36
bool has_mask_
Definition: websocket.h:625
rendered_template()
Definition: mustache.h:35
void shutdown_readwrite()
Definition: socket_adaptors.h:58
A base class for websocket connection.
Definition: websocket.h:22
Definition: middleware.h:27
bool close_connection
Whether or not the server should shut down the TCP connection once a response is sent.
Definition: parser.h:187
std::function< void(request &, response &, const routing_params &)> erased_handler_
Definition: routing.h:552
std::atomic< unsigned int > & queue_length_
Definition: http_connection.h:634
void on_tick()
Definition: http_server.h:50
A class to represent any data coming after the ? in the request URL into key-value pairs.
Definition: query_string.h:291
A websocket connection.
Definition: websocket.h:64
std::tuple< Middlewares... > * middlewares_
Definition: http_server.h:245
A rule dealing with websockets.
Definition: routing.h:371
rvalue load_nocopy_internal(char *data, size_t size)
Definition: json.h:819
bool completed_
Definition: http_response.h:267
H1 & handler
Definition: routing.h:111
bool is_static_type()
Check whether the response has a static file defined.
Definition: http_response.h:210
virtual void close(const std::string &msg="quit")=0
rvalue(const rvalue &r)
Definition: json.h:242
void set_base(const std::string &path)
Definition: mustache.h:667
rendered_template(std::string &body)
Definition: mustache.h:38
void handle_upgrade(const request &req, response &, SocketAdaptor &&adaptor) override
Definition: routing.h:389
int start
Definition: mustache.h:62
void do_accept()
Definition: http_server.h:194
size_t & stream_threshold()
Get the response body size (in bytes) beyond which Crow automatically streams responses.
Definition: app.h:193
wvalue(list &r)
Definition: json.h:1331
bool ssl_used() const
Definition: app.h:402
std::string render_string() const
Output a returnable template from this mustache template.
Definition: mustache.h:383
Definition: http_response.h:25
bool operator()(const std::string &l, const std::string &r) const
Definition: ci_map.h:29
bool operator>(const r_string &l, const std::string &r)
Definition: json.h:201
std::string render_string(context &ctx) const
Apply the values from the context provided and output a returnable template from this mustache templa...
Definition: mustache.h:395
num_type nt
The specific type of the number if t_ is a number.
Definition: json.h:1251
friend std::enable_if<(N< std::tuple_size< typename std::remove_reference< Container >::type >::value), bool >::type middleware_call_helper(Container &middlewares, request &req, response &res, Context &ctx)
Definition: middleware.h:127
void handle(request &, response &res, const routing_params &) override
Definition: routing.h:383
bool keep_alive
Definition: http_request.h:34
@ TOO_MANY_REQUESTS
Definition: common.h:201
void operator()(F cparams)
Definition: routing.h:154
wvalue & operator=(std::function< std::string(std::string &)> &&func)
Definition: json.h:1645
std::vector< std::string > write_buffers_
Definition: websocket.h:614
size_t get_size(Node *node)
Definition: routing.h:999
const std::string dd
Definition: multipart.h:18
std::vector< Blueprint * > blueprints_
Definition: routing.h:1155
r_string & operator=(r_string &&r)
Definition: json.h:141
bool operator==(const r_string &l, const r_string &r)
Definition: json.h:206
std::string url
The endpoint without any parameters.
Definition: http_request.h:28
typename black_magic::has_type< MW, typename App::mw_container_t > check_app_contains
Definition: middleware.h:303
detail::r_string s() const
The string value.
Definition: json.h:398
CatchallRule & catchall_rule()
Definition: routing.h:1186
Definition: middleware.h:80
@ RANGE_NOT_SATISFIABLE
Definition: common.h:198
Definition: middleware.h:56
A wrapper for the asio::ip::tcp::socket and asio::ssl::stream.
Definition: socket_adaptors.h:18
virtual void send_ping(const std::string &msg)=0
self_t & timeout(std::uint8_t timeout)
Set the connection timeout in seconds (default is 5)
Definition: app.h:132
wvalue(std::int32_t value)
Definition: json.h:1296
WebSocketRule & websocket()
Definition: routing.h:451
bool is_cached() const
Definition: json.h:649
black_magic::S< typename black_magic::promote_t< Args >... > args_type
Definition: routing.h:243
CatchallRule & catchall_route()
Create a route for any requests without a proper route (Use CROW_CATCHALL_ROUTE instead)
Definition: app.h:100
friend std::ostream & operator<<(std::ostream &os, const rvalue &r)
Definition: json.h:720
void operator()(Func f)
Definition: routing.h:505
self_t & tick(Duration d, Func f)
Set a custom duration and function to run on every tick.
Definition: app.h:206
int end
Definition: mustache.h:63
Func f
Definition: routing.h:205
type t() const
Definition: json.h:1247
void set_tick_function(std::chrono::milliseconds d, std::function< void()> f)
Definition: http_server.h:44
static const bool value
Definition: middleware.h:200
CORSHandler is a global middleware for setting CORS headers.
Definition: cors.h:132
wvalue(std::int8_t value)
Definition: json.h:1292
std::string prefix_
Definition: routing.h:1150
std::function< bool(const crow::request &)> accept_handler_
Definition: websocket.h:638
std::unordered_map< std::string, std::string > get_dict(const std::string &name) const
Works similar to get_list() except the brackets are mandatory must not be empty.
Definition: query_string.h:439
Definition: middleware.h:59
A wrapper for nodejs/http-parser.
Definition: parser.h:18
boost::asio::io_service * io_service
Definition: http_request.h:38
@ EXPECTATION_FAILED
Definition: common.h:199
wvalue(double value)
Definition: json.h:1303
Definition: middleware.h:51
void operator()(F cparams)
Definition: routing.h:144
std::string remote_ip_address
The IP address from which the request was sent.
Definition: http_request.h:32
A search tree.
Definition: routing.h:628
detail::context< Middlewares... > ctx_
Definition: http_connection.h:627
void optimize()
Definition: routing.h:662
const int RULE_SPECIAL_REDIRECT_SLASH
Definition: routing.h:624
rvalue load(const char *data, size_t size)
Definition: json.h:1204
const std::string & get_header_value(const T &headers, const std::string &key)
Find and return the value associated with the key. (returns an empty string if nothing is found)
Definition: http_request.h:13
ParamType param
Definition: routing.h:637
const char cr
Definition: common.h:11
char * end_
Definition: json.h:709
void do_write_general()
Definition: http_connection.h:415
int header_building_state
Definition: parser.h:180
Router router_
Definition: app.h:442
const rvalue & operator[](int index) const
Definition: json.h:559
self_t & name(std::string name) noexcept
Definition: routing.h:458
Definition: middleware.h:35
const char lf
Definition: common.h:12
friend rvalue load(const char *data, size_t size)
Definition: json.h:1204
std::string build_header(int opcode, size_t size)
Generate the websocket headers using an opcode and the message size (in bytes).
Definition: websocket.h:200
void copy_l(const rvalue &r)
Definition: json.h:657
std::vector< detail::task_timer * > task_timer_pool_
Definition: http_server.h:228
void userdata(void *u)
Definition: websocket.h:32
identifier_type highest_id_
Definition: task_timer.h:130
Blueprint(Blueprint &&value)
Definition: routing.h:1054
std::string date_str_
Definition: http_connection.h:615
~r_string()
Definition: json.h:125
std::vector< char * > key_value_pairs_
Definition: query_string.h:485
Definition: routing.h:174
BaseRule(std::string rule)
Definition: routing.h:33
message(const request &req)
Create a multipart message from a request data.
Definition: multipart.h:140
response(returnable &value)
Definition: http_response.h:76
An HTTP request.
Definition: http_request.h:24
void handle_header()
Definition: http_connection.h:97
void before_handle(request &, response &, context &)
Definition: utf-8.h:13
std::vector< std::unique_ptr< BaseRule > > all_rules_
Definition: routing.h:1153
wvalue & operator=(int value)
Definition: json.h:1505
WebSocketReadState state_
Definition: websocket.h:620
response(std::string contentType, std::string body)
Definition: http_response.h:93
constexpr number(std::int64_t value) noexcept
Definition: json.h:1263
static std::true_type f(typename check_before_handle_arity_3_const< T >::template get< C > *)
self_t & onclose(Func f)
Definition: routing.h:415
void operator()(crow::request &req, crow::response &res, Args &&... args) const
Definition: middleware.h:267
wvalue & operator=(long value)
Definition: json.h:1496
uint64_t ui
Definition: json.h:1256
void handle(request &req, response &res)
Process the request and generate a response for it.
Definition: app.h:72
void operator()(std::string name, Func &&f)
Definition: routing.h:545
wvalue(std::uint64_t value)
Definition: json.h:1289
static std::true_type f(typename check_after_handle_arity_3_const< T >::template get< C > *)
static int on_body(http_parser *self_, const char *at, size_t length)
Definition: parser.h:79
int pos
Definition: mustache.h:64
Trie()
Definition: routing.h:653
constexpr number(double value) noexcept
Definition: json.h:1265
invalid_template_exception(const std::string &msg)
Definition: mustache.h:23
void process_tasks()
Definition: task_timer.h:89
DynamicRule & route_dynamic(std::string &&rule)
Create a dynamic route using a rule (Use CROW_ROUTE instead)
Definition: app.h:78
void close()
Definition: socket_adaptors.h:52
response res
Definition: http_connection.h:607
void tick_handler(const boost::system::error_code &ec)
Definition: task_timer.h:111
static ILogHandler *& get_handler_ref()
Definition: logging.h:136
uint16_t concurrency_
Definition: app.h:437
void log(std::string message, LogLevel level) override
Definition: logging.h:42
void signal_clear()
Definition: http_server.h:169
bool server_started_
Definition: app.h:464
void parse()
Definition: mustache.h:406
size_t res_stream_threshold_
Definition: http_connection.h:632
static const int error_bit
Definition: json.h:225
uint8_t owned_
Definition: json.h:174
Blueprint(const std::string &prefix)
Definition: routing.h:1032
The main server application.
Definition: app.h:47
CatchallRule catchall_rule_
Definition: routing.h:1154
@ PERMANENT_REDIRECT
Definition: common.h:186
int code
The Status code for the response.
Definition: http_response.h:37
self_t & onmessage(Func f)
Definition: routing.h:408
wvalue & operator=(unsigned int value)
Definition: json.h:1532
int64_t i() const
The integer value.
Definition: json.h:345
r_string(char *s, char *e)
Definition: json.h:123
CORSRules & max_age(int max_age)
Set Access-Control-Max-Age. Default is none.
Definition: cors.h:55
void set_connection_parameters()
Definition: parser.h:156
std::unordered_map< std::string, std::string > pop_dict(const std::string &name)
Works the same as get_dict() but removes the values from the query string.
Definition: query_string.h:455
const char * const_iterator
Definition: json.h:170
std::function< void(request &, response &, const routing_params &)> wrap(Func f, black_magic::seq< Indices... >)
Definition: routing.h:524
self_t & bindaddr(std::string bindaddr)
The IP address that Crow will handle requests on (default is 0.0.0.0)
Definition: app.h:146
std::vector< rvalue > lo()
The list or object value.
Definition: json.h:409
std::function< void(crow::websocket::connection &)> error_handler_
Definition: routing.h:439
static_file_info file_info
Definition: http_response.h:270
HTTPParser< Connection > parser_
Definition: http_connection.h:605
Definition: mustache.h:33
char * start_
Definition: json.h:708
std::string bindaddr_
Definition: http_server.h:239
F f
Definition: middleware.h:296
std::string execute(std::string txt="") const
Definition: json.h:1696
void debug_print()
Definition: routing.h:1575
std::function< void(crow::websocket::connection &, const std::string &, bool)> message_handler_
Definition: routing.h:437
const header & get_header_object(const T &headers, const std::string &key)
Same as get_header_value_object() but for multipart::header.
Definition: multipart.h:47
wvalue(std::string const &value)
Definition: json.h:1309
query_string url_params
What comes after the ? in the URL.
Definition: parser.h:184
CORSRules default_
Definition: cors.h:182
void escape(const std::string &in, std::string &out) const
Definition: mustache.h:153
static std::string base64decode(const char *data, size_t size)
Definition: utility.h:585
std::vector< std::pair< std::string, CORSRules > > rules
Definition: cors.h:181
std::string templates_dir_
Definition: routing.h:1152
size_t identifier_type
Definition: task_timer.h:21
wvalue(bool value)
Definition: json.h:1280
self_t & methods(HTTPMethod method)
Definition: routing.h:464
friend rvalue load_nocopy_internal(char *data, size_t size)
Definition: json.h:819
response(int code, std::string body)
Definition: http_response.h:69
void internal_add_rule_object(const std::string &rule, BaseRule *ruleObject, const uint16_t &BP_index, std::vector< Blueprint * > &blueprints)
Definition: routing.h:1191
bool operator==(const Blueprint &value)
Definition: routing.h:1069
Definition: routing.h:272
Connection(const crow::request &req, Adaptor &&adaptor, std::function< void(crow::websocket::connection &)> open_handler, std::function< void(crow::websocket::connection &, const std::string &, bool)> message_handler, std::function< void(crow::websocket::connection &, const std::string &)> close_handler, std::function< void(crow::websocket::connection &)> error_handler, std::function< bool(const crow::request &)> accept_handler)
Constructor for a connection.
Definition: websocket.h:72
@ INTERNAL_SERVER_ERROR
Definition: common.h:204
Blueprint & operator=(Blueprint &&value) noexcept
Definition: routing.h:1061
type t_
Definition: json.h:714
std::vector< char * > pop_list(const std::string &name, bool use_brackets=true)
Similar to get_list() but it removes the.
Definition: query_string.h:416
wvalue & operator[](unsigned index)
Definition: json.h:1653
void handle(request &req, response &res, const routing_params ¶ms) override
Definition: routing.h:495
std::vector< std::string > sending_buffers_
Definition: websocket.h:613
uint16_t blueprint_index
Definition: routing.h:635
std::string server_name_
Definition: app.h:439
void do_write()
Definition: http_connection.h:521
TaggedRule(std::string rule)
Definition: routing.h:562
std::vector< std::function< std::string()> > get_cached_date_str_pool_
Definition: http_server.h:229
void start(std::string &&hello)
Send the HTTP upgrade response.
Definition: websocket.h:227
wvalue(wvalue &&r)
Definition: json.h:1416
const rvalue & operator[](size_t index) const
Definition: json.h:570
bool is_completed() const noexcept
Check if the response has completed (whether response.end() has been called)
Definition: http_response.h:118
static void setHandler(ILogHandler *handler)
Definition: logging.h:125
virtual void handle_upgrade(const request &, response &res, SocketAdaptor &&)
Definition: routing.h:49
std::vector< wvalue > list
Definition: json.h:1245
bool is_open()
Definition: socket_adaptors.h:47
void dispatch(CompletionHandler handler)
Send data through the socket.
Definition: websocket.h:110
template_t compile(const std::string &body)
Definition: mustache.h:623
std::function< bool(const crow::request &)> accept_handler_
Definition: routing.h:440
@ NOT_FOUND
Definition: common.h:191
void ignore()
Ignore CORS and don't send any headers.
Definition: cors.h:69
std::string dump() const override
Represent all parts as a string (does not include message headers)
Definition: multipart.h:92
void operator()(const request &req, response &res, Args... args)
Definition: routing.h:199
r_string(const r_string &r)
Definition: json.h:131
tcp::socket socket_
Definition: socket_adaptors.h:82
virtual std::string dump() const =0
std::function< void()> tick_function_
Definition: http_server.h:243
void handle_upgrade(const request &req, response &res, Adaptor &&adaptor)
Process an Upgrade request.
Definition: app.h:66
void clear()
Definition: parser.h:129
wvalue(std::uint32_t value)
Definition: json.h:1287
uint32_t methods_
Definition: routing.h:83
std::string rule_
Definition: routing.h:85
T type
Definition: routing.h:104
template_t load(const std::string &filename)
Definition: mustache.h:706
void add_header(std::string key, std::string value)
Definition: http_request.h:50
HTTP response.
Definition: http_response.h:29
std::string body_
Definition: mustache.h:620
Connection(boost::asio::io_service &io_service, Handler *handler, const std::string &server_name, std::tuple< Middlewares... > *middlewares, std::function< std::string()> &get_cached_date_str_f, detail::task_timer &task_timer, typename Adaptor::context *adaptor_ctx_, std::atomic< unsigned int > &queue_length)
Definition: http_connection.h:39
Definition: middleware.h:48
void render_internal(int actionBegin, int actionEnd, std::vector< context * > &stack, std::string &out, int indent) const
Definition: mustache.h:199
uint8_t option_
Definition: json.h:716
std::string boundary
The text boundary that separates different parts
Definition: multipart.h:77
Server< Crow, SocketAdaptor, Middlewares... > server_t
The HTTP server.
Definition: app.h:53
virtual ~returnable()
Definition: returnable.h:17
void operator()(request &req, response &res, const routing_params ¶ms)
Definition: routing.h:255
@ CONTINUE
Definition: common.h:169
std::function< void(const crow::request &, crow::response &)> handler_
Definition: routing.h:363
uint16_t port_
Definition: app.h:436
Server(Handler *handler, std::string bindaddr, uint16_t port, std::string server_name=std::string("Crow/")+VERSION, std::tuple< Middlewares... > *middlewares=nullptr, uint16_t concurrency=1, uint8_t timeout=5, typename Adaptor::context *adaptor_ctx=nullptr)
Definition: http_server.h:29
std::string raw_url
The full URL containing the ? and URL parameters.
Definition: http_request.h:27
wvalue & operator=(std::nullptr_t)
Definition: json.h:1445
constexpr const char * method_strings[]
Definition: common.h:110
std::string get_cookie(const std::string &key) const
Definition: cookie_parser.h:38
Definition: middleware_context.h:14
JSON write value.
Definition: json.h:1233
num_type
Definition: json.h:106
Crow self_t
This crow application.
Definition: app.h:51
DynamicRule & new_rule_dynamic(const std::string &rule)
Definition: routing.h:1167
bool operator<(const r_string &l, const r_string &r)
Definition: json.h:191
bool IsSimpleNode() const
Definition: routing.h:640
void set_error()
Definition: json.h:623
void check_destroy()
Definition: http_connection.h:567
static int on_header_field(http_parser *self_, const char *at, size_t length)
Definition: parser.h:32
uint32_t get_methods()
Definition: routing.h:62
identifier_type schedule(const task_type &task, std::uint8_t timeout)
Schedule the given task to be executed after the given time.
Definition: task_timer.h:71
detail::r_string key_
Definition: json.h:710
typename black_magic::pop_back< Middlewares... >::template rebind<::crow::detail::partial_context > parent_context
Definition: middleware_context.h:16
std::function< std::string(std::string &)> f
Value if type is a function (C++ lambda)
Definition: json.h:1271
CORSRules & global()
Get the global CORS policy.
Definition: cors.h:161
std::uint8_t default_timeout_
Definition: task_timer.h:123
A base class for all rules.
Definition: routing.h:30
mph_map headers
(optional) The first part before the data, Contains information regarding the type of data and encodi...
Definition: multipart.h:58
std::string body_
Definition: mustache.h:41
void clear()
Definition: query_string.h:346
clock_type::time_point time_type
Definition: task_timer.h:25
void after_handle(request &, response &res, context &)
Definition: utf-8.h:16
int count(const std::string &str)
Definition: json.h:1665
void set_global_base(const std::string &path)
Definition: mustache.h:678
bool isTagInsideObjectBlock(const int ¤t, const std::vector< context * > &stack) const
Definition: mustache.h:173
@ PRECONDITION_REQUIRED
Definition: common.h:200
std::string load_text(const std::string &filename)
Definition: mustache.h:694
ParamType
Definition: common.h:214
boost::asio::deadline_timer tick_timer_
Definition: http_server.h:232
void get_recursive_child_methods(Blueprint *blueprint, std::vector< HTTPMethod > &methods)
Definition: routing.h:1225
auto find_context(const std::string &name, const std::vector< context * > &stack, bool shouldUseOnlyFirstStackValue=false) const -> std::pair< bool, context & >
Definition: mustache.h:88
std::string dump() const
Definition: json.h:1890
size_t estimate_length() const
Returns an estimated size of the value in bytes.
Definition: json.h:1712
wvalue(float value)
Definition: json.h:1301
std::function< void(crow::websocket::connection &)> open_handler_
Definition: websocket.h:634
std::function< void(const crow::request &, crow::response &, Args...)> type
Definition: routing.h:235
One part of the multipart message.
Definition: multipart.h:56
Used for tuning CORS policies.
Definition: cors.h:11
wvalue(char const *value)
Definition: json.h:1306
HTTPParser(Handler *handler)
Definition: parser.h:96
std::function< void(crow::websocket::connection &)> open_handler_
Definition: routing.h:436
wvalue()
Definition: json.h:1274
uint16_t lremain_
Definition: json.h:713
void do_write()
Send the buffers' data through the socket.
Definition: websocket.h:568
void write(const std::string &body_part)
Definition: http_response.h:172
void run()
Definition: http_server.h:61
wvalue & operator=(unsigned long long value)
Definition: json.h:1514
void apply(crow::response &res)
Set response headers.
Definition: cors.h:105
self_t & onopen(Func f)
Definition: routing.h:401
CORSRules & global()
Global CORS policy.
Definition: cors.h:195
DynamicRule(std::string rule)
Definition: routing.h:483
returnable(std::string ctype)
Definition: returnable.h:13
void debug_node_print(Node *node, int level)
Definition: routing.h:695
r_string()
Definition: json.h:122
~task_timer()
Definition: task_timer.h:36
char * get(const std::string &name) const
Get a value from a name, used for ?name=value.
Definition: query_string.h:369
@ NON_AUTHORITATIVE_INFORMATION
Definition: common.h:175
Handles matching requests to existing rules and upgrade requests.
Definition: routing.h:1161
void moved(const std::string &location)
Return a "Found (Moved Temporarily)" response.
Definition: http_response.h:156
const header & get_header_object(const std::string &key) const
Definition: multipart.h:64
void redirect_perm(const std::string &location)
Return a "Permanent Redirect" response.
Definition: http_response.h:146
response(int code, std::string contentType, std::string body)
Definition: http_response.h:99
black_magic::S< typename black_magic::promote_t< Args >... > args_type
Definition: routing.h:250
void validate()
Definition: routing.h:1277
std::function< void(crow::websocket::connection &, const std::string &)> close_handler_
Definition: routing.h:438
void * middleware_context
Definition: http_request.h:36
constexpr number() noexcept
Definition: json.h:1259
std::vector< std::unique_ptr< BaseRule > > all_rules_
Definition: routing.h:1602
Node head_
Definition: routing.h:1021
wvalue & operator=(unsigned long value)
Definition: json.h:1523
std::string default_loader(const std::string &filename)
Definition: mustache.h:643
std::string headers_
Definition: cors.h:119
bool operator==(const rvalue &l, const std::string &r)
Definition: json.h:778
bool ignore_
Definition: cors.h:115
template_t load_unsafe(const std::string &filename)
Definition: mustache.h:713
static constexpr bool value
Definition: middleware.h:263
std::string dump() const override
Definition: mustache.h:43
std::unique_ptr< rvalue[]> l_
Definition: json.h:711
void dispatch(CompletionHandler handler)
Send data to whoever made this request with a completion handler.
Definition: http_request.h:74
double d
Definition: json.h:1254
void set_(Func f, typename std::enable_if<!std::is_same< typename std::tuple_element< 0, std::tuple< Args..., void >>::type, const request & >::value, int >::type=0)
Definition: routing.h:177
size_t res_stream_threshold_
Definition: app.h:441
std::string dump(int part_) const
Represent an individual part as a string.
Definition: multipart.h:107
static const int MAX_KEY_VALUE_PAIRS_COUNT
Definition: query_string.h:294
boost::array< char, 4096 > buffer_
Definition: websocket.h:616
boost::asio::io_service & io_service_
Definition: task_timer.h:124
static const int cached_bit
Definition: json.h:224
Definition: routing.h:631
detail::context< Middlewares... > context_t
Definition: app.h:409
uint16_t remaining_length16_
Definition: websocket.h:621
wvalue(object &&value)
Definition: json.h:1319
static std::string timestamp()
Definition: logging.h:67
std::string header_value
Definition: parser.h:182
CORSRules & prefix(const std::string &prefix)
Handle CORS on a specific prefix path.
Definition: cors.h:147
virtual ~connection()
Definition: websocket.h:30
self_t & port(std::uint16_t port)
Set the port that Crow will handle requests on.
Definition: app.h:118
void signal_add(int signal_number)
Definition: http_server.h:174
void before_handle(crow::request &, crow::response &, context &)
Definition: cors.h:137
tcp::endpoint remote_endpoint()
Definition: socket_adaptors.h:42
void start_deadline()
Definition: http_connection.h:584
virtual void send_binary(const std::string &msg)=0
rvalue * begin() const
Definition: json.h:526
@ NO_CONTENT
Definition: common.h:176
A class for scheduling functions to be called after a specific amount of ticks. A tick is equal to 1 ...
Definition: task_timer.h:17
void clear()
Definition: http_response.h:123
T & get_middleware()
Definition: app.h:420
int statResult
Definition: http_response.h:223
std::string load_text_unsafe(const std::string &filename)
Definition: mustache.h:701
static int on_url(http_parser *self_, const char *at, size_t length)
Definition: parser.h:26
char * s_
Start.
Definition: json.h:172
@ CONFLICT
Definition: common.h:194
@ RESET_CONTENT
Definition: common.h:177
std::mutex start_mutex_
Definition: app.h:466
uint64_t remaining_length_
Definition: websocket.h:622
void moved_perm(const std::string &location)
Return a "Moved Permanently" response.
Definition: http_response.h:166
std::function< void()> complete_request_handler_
Definition: http_response.h:268
uint16_t mini_header_
Definition: websocket.h:627
bool is_FIN()
Check if the FIN bit is set.
Definition: websocket.h:467
void complete_request()
Call the after handle middleware and send the write the response to the connection.
Definition: http_connection.h:203
A mustache template object.
Definition: mustache.h:73
void set_cookie(const std::string &key, const std::string &value)
Definition: cookie_parser.h:46
rvalue(type t) noexcept
Definition: json.h:232
std::function< void(const crow::request &, crow::response &, Args...)> type
Definition: routing.h:242
bool error() const
Definition: json.h:628
black_magic::S< typename black_magic::promote_t< Args >... > args_type
Definition: routing.h:236
wvalue & operator=(const char *str)
Definition: json.h:1541
wvalue & operator=(bool value)
Definition: json.h:1450
bool is_reading
Definition: websocket.h:624
LogLevel
Definition: logging.h:14
std::function< void(crow::websocket::connection &)> error_handler_
Definition: websocket.h:637
std::unordered_multimap< std::string, part, ci_hash, ci_key_eq > mp_map
Multipart map (key is the name parameter).
Definition: multipart.h:71
@ TEMPORARY_REDIRECT
Definition: common.h:185
std::string & get_global_template_base_directory_ref()
A base directory not related to any blueprint.
Definition: mustache.h:636
void emplace_back(rvalue &&v)
Definition: json.h:667
asio::ip::tcp tcp
Definition: http_connection.h:25
T::context & get_context(const request &req)
Definition: app.h:412
void run()
Run the server.
Definition: app.h:277
wvalue & operator=(object &&value)
Definition: json.h:1630
void post(CompletionHandler handler)
Send data to whoever made this request with a completion handler and return immediately.
Definition: http_request.h:67
rvalue(type t, char *s, char *e) noexcept
Definition: json.h:236
std::tuple< Middlewares... > mw_container_t
Definition: app.h:410
std::vector< Node * > children
Definition: routing.h:638
wvalue & operator=(short value)
Definition: json.h:1478
unsigned char http_ver_minor
Definition: http_request.h:33
std::string static_dir_
Definition: routing.h:1151
virtual void validate()=0
void do_read()
Read a websocket message.
Definition: websocket.h:250
std::string get_remote_ip() override
Definition: websocket.h:193
typename std::conditional< N==sizeof...(Middlewares) - 1, partial_context, typename parent_context::template partial< N > >::type partial
Definition: middleware_context.h:18
self_t & methods(HTTPMethod method, MethodArgs... args_method)
Definition: routing.h:471
Crow()
Definition: app.h:58
void * userdata()
Definition: websocket.h:33
void debug_print()
Definition: routing.h:731
CORSRules & methods(crow::HTTPMethod method, Methods... method_list)
Set Access-Control-Allow-Methods. Default is "*".
Definition: cors.h:31
static const int pos
Definition: routing.h:105
void dump_string(const std::string &str, std::string &out) const
Definition: json.h:1755
std::enable_if< black_magic::is_callable< F, const crow::request, crow::response &, Args... >::value >::type wrapped_handler_call(crow::request &req, crow::response &res, const F &f, Args &&... args)
Definition: middleware.h:206
Blueprint & operator=(const Blueprint &value)=delete
std::string custom_templates_base
Definition: routing.h:78
std::enable_if<(N< std::tuple_size< typename std::remove_reference< Container >::type >::value), bool >::type middleware_call_helper(Container &middlewares, request &req, response &res, Context &ctx)
Definition: middleware.h:127
void start()
Definition: http_connection.h:80
tcp::socket & raw_socket()
Get the TCP socket handling data trasfers, regardless of what layer is handling transfers on top of t...
Definition: socket_adaptors.h:31
~Connection()
Definition: http_connection.h:64
void send_ping(const std::string &msg) override
Send a "Ping" message.
Definition: websocket.h:126
Definition: middleware.h:192
void validate_bp(std::vector< Blueprint * > blueprints)
Definition: routing.h:1247
virtual void send_text(const std::string &msg)=0
std::unique_ptr< server_t > server_
Definition: app.h:460
Router()
Definition: routing.h:1164
void close(const std::string &msg) override
Send a close signal.
Definition: websocket.h:176
const O & get_header_value_object(const T &headers, const std::string &key)
Find and return the value object associated with the key. (returns an empty class if nothing is found...
Definition: multipart.h:35
void handle(request &req, response &res, const routing_params ¶ms) override
Definition: routing.h:595
response(std::string body)
Definition: http_response.h:68
bool allow_credentials_
Definition: cors.h:121
size_t size() const
Definition: json.h:167
response()
Definition: http_response.h:66
std::string content_type
Definition: returnable.h:10
ci_map headers
The request/response headers.
Definition: multipart.h:76
void add_header(std::string key, std::string value)
Add a new header to the response.
Definition: http_response.h:55
static const bool value
Definition: middleware.h:76
void render_fragment(const std::pair< int, int > fragment, int indent, std::string &out) const
Definition: mustache.h:343
An abstract class that allows any other class to be returned by a handler.
Definition: returnable.h:8
bool operator!=(const rvalue &l, const std::string &r)
Definition: json.h:788
void parse_body(std::string body, std::vector< part > §ions, mp_map &part_map)
Definition: multipart.h:167
@ BAD_REQUEST
Definition: common.h:188
self_t & ssl(T &&)
Definition: app.h:392
black_magic::arguments< N >::type::template rebind< TaggedRule > & new_rule_tagged(std::string &&rule)
Definition: routing.h:1101
ci_map headers
Definition: parser.h:183
rvalue & operator=(const rvalue &r)
Definition: json.h:253
bool manual_length_header
Whether Crow should automatically add a "Content-Length" header.
Definition: http_response.h:45
Definition: routing.h:102
const char * get_type_str(type t)
Definition: json.h:91
bool b() const
The boolean value.
Definition: json.h:388
wvalue(std::uint8_t value)
Definition: json.h:1283
const std::string crlf("\r\n")
HTTPMethod method
Definition: http_request.h:26
auto route(std::string &&rule) -> typename std::result_of< decltype(&Router::new_rule_tagged< Tag >)(Router, std::string &&)>::type
Create a route using a rule (Use CROW_ROUTE instead)
Definition: app.h:88
void register_blueprint(Blueprint &blueprint)
Definition: routing.h:1114
std::vector< BaseRule * > rules
Definition: routing.h:1594
CORSRules & methods(crow::HTTPMethod method)
Set Access-Control-Allow-Methods. Default is "*".
Definition: cors.h:23
type t() const
The type of the JSON value.
Definition: json.h:321
std::string origin_
Definition: cors.h:117
wvalue & operator=(long long value)
Definition: json.h:1487
std::string res_body_copy_
Definition: http_connection.h:616
int qs_strncmp(const char *s, const char *qs, size_t n)
Definition: query_string.h:51
wvalue(std::nullptr_t)
Definition: json.h:1277
std::map< identifier_type, std::pair< time_type, task_type > > tasks_
Definition: task_timer.h:126
static int on_header_value(http_parser *self_, const char *at, size_t length)
Definition: parser.h:51
Definition: cookie_parser.h:33
CORSRules & headers(const std::string &header, Headers... header_list)
Set Access-Control-Allow-Headers. Default is "*".
Definition: cors.h:47
wvalue(object const &value)
Definition: json.h:1317
Hashing function for ci_map (unordered_multimap).
Definition: ci_map.h:10
CORSRules & find_rule(const std::string &path)
Definition: cors.h:167
size_t size() const
Definition: json.h:548
void dump_internal(const wvalue &v, std::string &out) const
Definition: json.h:1762
Definition: routing.h:1592
wvalue(std::int16_t value)
Definition: json.h:1294
WebSocketRule(std::string rule)
Definition: routing.h:376
T::context & get()
Definition: middleware_context.h:21
static int on_message_complete(http_parser *self_)
Definition: parser.h:85
boost::asio::signal_set signals_
Definition: http_server.h:231
detail::task_timer::identifier_type task_id_
Definition: http_connection.h:618
virtual ~BaseRule()
Definition: routing.h:37
black_magic::arguments< N >::type::template rebind< TaggedRule > & new_rule_tagged(const std::string &rule)
Definition: routing.h:1176
@ UNAVAILABLE_FOR_LEGAL_REASONS
Definition: common.h:202
std::vector< std::pair< int, int > > fragments_
Definition: mustache.h:618
std::uint8_t get_default_timeout() const
Get the default timeout. (Default: 5)
Definition: task_timer.h:86
response & operator=(response &&r) noexcept
Definition: http_response.h:107
std::vector< std::string > keys() const
Definition: json.h:1684
std::string trim(std::string &string, const char &excess='"') const
Definition: multipart.h:251
CatchallRule catchall_rule_
Definition: routing.h:1590
@ UNAUTHORIZED
Definition: common.h:189
void debug_print()
Print the routing paths defined for each HTTP method.
Definition: app.h:334
std::uint8_t timeout_
Definition: http_server.h:236
CORSRules & allow_credentials()
Enable Access-Control-Allow-Credentials.
Definition: cors.h:62
void handle()
Definition: http_connection.h:109
wvalue & operator=(std::initializer_list< std::pair< std::string const, wvalue >> initializer_list)
Definition: json.h:1592
const char * iterator
Definition: json.h:169
Allows the user to assign parameters using functions.
Definition: routing.h:448
std::function< void(crow::websocket::connection &, const std::string &, bool)> message_handler_
Definition: websocket.h:635
@ MULTIPLE_CHOICES
Definition: common.h:180
wvalue & operator=(object const &value)
Definition: json.h:1611
bool upgrade
Definition: http_request.h:34
std::string static_dir() const
Definition: routing.h:1084
std::unique_ptr< list > l
Value if type is a list.
Definition: json.h:1269
self_t & onerror(Func f)
Definition: routing.h:422
std::string tag_name(const Action &action) const
Definition: mustache.h:84
std::vector< Blueprint * > blueprints_
Definition: routing.h:1603
std::size_t size() const
If the wvalue is a list, it returns the length of the list, otherwise it returns 1.
Definition: json.h:1704
boost::optional< std::pair< std::string, std::string > > qs_dict_name2kv(const char *dict_name, char *const *qs_kv, int qs_kv_size, int nth=0)
Definition: query_string.h:202
rvalue() noexcept
Definition: json.h:228
std::chrono::milliseconds tick_interval_
Definition: app.h:449
std::future< void > run_async()
Non-blocking version of run()
Definition: app.h:311
request & req
Definition: routing.h:113
void do_write_static()
Definition: http_connection.h:385
@ FORBIDDEN
Definition: common.h:190
void end()
Set the response completion flag and call the handler (to send the response).
Definition: http_response.h:178
The parsed multipart request/response.
Definition: multipart.h:74
status
Definition: common.h:167
virtual void send_pong(const std::string &msg)=0
static void setLogLevel(LogLevel level)
Definition: logging.h:123
void optimizeNode(Node *node)
Definition: routing.h:672
Definition: mustache.h:20
char * qs_scanvalue(const char *key, const char *qs, char *val, size_t val_len)
Definition: query_string.h:245
@ OK
Definition: common.h:172
A blueprint can be considered a smaller section of a Crow app, specifically where the router is conec...
Definition: routing.h:1029
constexpr number(std::uint64_t value) noexcept
Definition: json.h:1261
uint64_t u() const
The unsigned integer value.
Definition: json.h:362
wvalue(const list &r)
Definition: json.h:1322
CORSRules & prefix(const std::string &prefix)
Handle CORS on specific prefix path.
Definition: cors.h:185
num_type nt() const
The number type of the JSON value.
Definition: json.h:333
friend std::ostream & operator<<(std::ostream &os, const query_string &qs)
Definition: query_string.h:352
std::string body
The actual data in the part.
Definition: multipart.h:59
Handler * handler_
Definition: http_server.h:234
wvalue(std::initializer_list< std::pair< std::string const, wvalue >> initializer_list)
Definition: json.h:1314
A read string implementation with comparison functionality.
Definition: json.h:120
std::unique_ptr< BaseRule > rule_to_upgrade_
Definition: routing.h:88
HTTPMethod
Definition: common.h:15
void send_pong(const std::string &msg) override
Send a "Pong" message.
Definition: websocket.h:140
void process_header()
Definition: parser.h:146
r_string & operator=(const r_string &r)
Definition: json.h:151
void set_(Func f, typename std::enable_if< std::is_same< typename std::tuple_element< 0, std::tuple< Args..., void >>::type, const request & >::value &&std::is_same< typename std::tuple_element< 1, std::tuple< Args..., void, void >>::type, response & >::value, int >::type=0)
Definition: routing.h:224
@ ACCEPTED
Definition: common.h:174
mp_map part_map
The individual parts of the message, organized in a map with the name header parameter being the key.
Definition: multipart.h:79
void operator()(F cparams)
Definition: routing.h:164
Definition: routing.h:233
bool check_version(unsigned char major, unsigned char minor) const
Definition: http_request.h:60
std::chrono::milliseconds tick_interval_
Definition: http_server.h:242
const std::string & get_header_value(const std::string &key) const
Definition: multipart.h:81
virtual void handle(request &, response &, const routing_params &)=0
void send_binary(const std::string &msg) override
Send a binary encoded message.
Definition: websocket.h:151
CORSRules & origin(const std::string &origin)
Set Access-Control-Allow-Origin. Default is "*".
Definition: cors.h:16
std::uint16_t port()
Get the port that Crow will handle requests on.
Definition: app.h:125
constexpr const char VERSION[]
Definition: version.h:5
std::string prefix() const
Definition: routing.h:1079
wvalue(const rvalue &r)
Create a write value from a read value (useful for editing JSON strings).
Definition: json.h:1342
std::vector< Blueprint * > & blueprints()
Definition: routing.h:1584
std::string method_name(HTTPMethod method)
Definition: common.h:156
req_handler_wrapper(Func f)
Definition: routing.h:194
@ MOVED_PERMANENTLY
Definition: common.h:181
std::string get_error(unsigned short code, std::tuple< uint16_t, std::vector< uint16_t >, routing_params > &found, const request &req, response &res)
Is used to handle errors, you insert the error code, found route, request, and response....
Definition: routing.h:1415
query_string & operator=(const query_string &qs)
Definition: query_string.h:310
self_t & concurrency(std::uint16_t concurrency)
Run the server on multiple threads using a specific number.
Definition: app.h:159
crow::detail::handler_call_bridge< TaggedRule< Args... >, App, Middlewares... > middlewares()
Enable local middleware for this handler.
Definition: routing.h:613
std::string & get_template_base_directory_ref()
Definition: mustache.h:629
std::unique_ptr< BaseRule > upgrade()
Definition: routing.h:41
detail::task_timer & task_timer_
Definition: http_connection.h:630
self_t & register_blueprint(Blueprint &blueprint)
Definition: app.h:198
DynamicRule & new_rule_dynamic(std::string &&rule)
Definition: routing.h:1089
uint32_t lsize_
Definition: json.h:712
@ METHOD_NOT_ALLOWED
Definition: common.h:192
void apply_blueprint(Blueprint &blueprint)
Definition: routing.h:1132
wvalue & operator=(const std::vector< T > &v)
Definition: json.h:1575
void stop()
Definition: http_server.h:162
const rvalue & operator[](const char *str) const
Definition: json.h:581
JSON read value.
Definition: json.h:222
Default rule created when CROW_ROUTE is called.
Definition: routing.h:557
wvalue(const wvalue &r)
Definition: json.h:1379
std::function< void()> tick_function_
Definition: app.h:450
bool operator!=(const Blueprint &value)
Definition: routing.h:1074
std::vector< Action > actions_
Definition: mustache.h:619
std::enable_if<(N< 0)>::type after_handlers_call_helper(Container &, Context &, request &, response &)
Definition: middleware.h:163
static int on_message_begin(http_parser *self_)
Definition: parser.h:20
static std::false_type f(typename check_global_call_false< MW >::template get< C > *)
std::function< void(crow::request &, crow::response &, Args...)> handler_
Definition: routing.h:621
int opcode()
Extract the opcode from the header.
Definition: websocket.h:473
Definition: routing.h:118
std::vector< std::string > keys() const
Definition: query_string.h:472
Definition: routing.h:192
self_t & ssl_file(T &&, Remain &&...)
Definition: app.h:381
CatchallRule & catchall_rule()
Definition: routing.h:1126
void after_handle(crow::request &req, crow::response &res, context &)
Definition: cors.h:140
Node * new_node(Node *parent)
Definition: routing.h:1012
@ PAYLOAD_TOO_LARGE
Definition: common.h:196
void set_header(std::string key, std::string value)
Set the value of an existing header in the response.
Definition: http_response.h:48
std::enable_if<!is_after_handle_arity_3_impl< MW >::value >::type after_handler_call(MW &mw, request &req, response &res, Context &ctx, ParentContext &)
Definition: middleware.h:111
~logger()
Definition: logging.h:99
std::string raw_url
Definition: parser.h:177
Definition: middleware.h:24
std::string max_age_
Definition: cors.h:120
std::string body
The actual payload containing the response data.
Definition: http_response.h:38
Handler * handler_
Definition: http_connection.h:601
std::vector< int > signals_
Definition: app.h:462